Aim:
In this article I will describe how to interface a Ultrasonic Range
Finder Module with a 8051 microcontroller. I will provide a HEX file
which you can burn into your 8051 directly to quickly test this whole
setup.
In this article I will describe how to interface a Ultrasonic Range
Finder Module with a 8051 microcontroller. I will provide a HEX file
which you can burn into your 8051 directly to quickly test this whole
setup.

Description:
We know that sound vibrations can not penetrate through solids. So what
happens is, when a source of sound generates vibrations they travel
through air at a speed of 220 meters per second. These vibrations when
they meet our ear we describe them as sound. As said earlier these
vibrations can not go through solid, so when they strike with a surface
like wall, they are reflected back at the same speed to the source,
which is called echo.
Ultrasonic sensor “HC-SR04” provides an output signal
proportional to distance based on the echo. The sensor here generates a
sound vibration in ultrasonic range upon giving a trigger, after that it
waits for the sound vibration to return. Now based on the parameters,
sound speed (220m/s) and time taken for the echo to reach the source, it
provides output pulse proportional to distance.
As shown in figure, at first we need to initiate the sensor for
measuring distance, that is a HIGH logic signal at trigger pin of sensor
for more than 10uS, after that a sound vibration is sent by sensor,
after a echo, the sensor provides a signal at the output pin whose width
is proportional to distance between source and obstacle.
We know that sound vibrations can not penetrate through solids. So what
happens is, when a source of sound generates vibrations they travel
through air at a speed of 220 meters per second. These vibrations when
they meet our ear we describe them as sound. As said earlier these
vibrations can not go through solid, so when they strike with a surface
like wall, they are reflected back at the same speed to the source,
which is called echo.
Ultrasonic sensor “HC-SR04” provides an output signal
proportional to distance based on the echo. The sensor here generates a
sound vibration in ultrasonic range upon giving a trigger, after that it
waits for the sound vibration to return. Now based on the parameters,
sound speed (220m/s) and time taken for the echo to reach the source, it
provides output pulse proportional to distance.
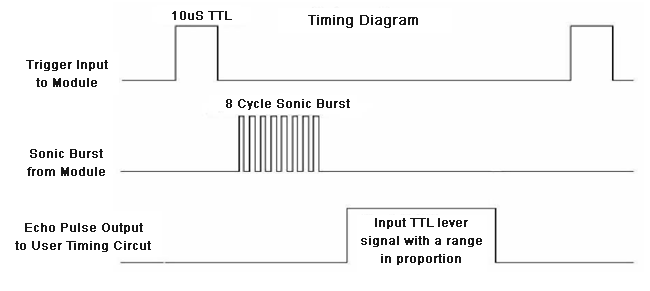
As shown in figure, at first we need to initiate the sensor for
measuring distance, that is a HIGH logic signal at trigger pin of sensor
for more than 10uS, after that a sound vibration is sent by sensor,
after a echo, the sensor provides a signal at the output pin whose width
is proportional to distance between source and obstacle.
Block Diagram

Schematic

Code
// ******************************************************
// Project: Ultrasonic sensor using 8051
// Author: Code Bloges
// Module description: Operate ultrasonic sensor
// ******************************************************
#include<REGX52.h>
#define port P2
#define dataport P0
int cms;
sbit trig=P3^5;
sbit rs=port^0;
sbit rw=port^1;
sbit e=port^2;
void delay(unsigned int msec)
{
int i,j;
for(i=0;i<msec;i++)
for(j=0;j<1275;j++);
}
void lcd_cmd(unsigned char item) // Function to send command to LCD
{
dataport = item;
rs= 0;
rw=0;
e=1;
delay(1);
e=0;
return;
}
void lcd_data(unsigned char item) // Function to send data to LCD
{
dataport = item;
rs= 1;
rw=0;
e=1;
delay(1);
e=0;
return;
}
void lcd_data_string(unsigned char *str) // Function to send string to LCD
{
int i=0;
while(str[i]!='\0')
{
lcd_data(str[i]);
i++;
delay(1);
}
return;
}
void send_pulse(void)
{
TH0=0x00;TL0=0x00;
trig=1; //Sending trigger pulse
delay(5); //Wait for about 10us
trig=0; //Turn off trigger
}
unsigned int get_range(void)
{
long int timer_val;
send_pulse();
while(!INT0); //Waiting until echo pulse is detected
while(INT0); //Waiting until echo pulse changes its state
timer_val=(TH0<<8)+TL0;
lcd_cmd(0x81);
lcd_data_string("output:");
lcd_cmd(0x8a);
if(timer_val<38000)
{
cms=timer_val/59;
if (cms!=0)
{
lcd_data(cms+48);
}
}
else
{
lcd_cmd(0x06);
lcd_data_string("Object out of range");
}
return cms;
}
void main()
{
lcd_cmd(0x38);
lcd_cmd(0x0c);
delay(2);
lcd_cmd(0x01);
delay(2);
lcd_cmd(0x81);
delay(2);
lcd_data_string("start");
delay(20);
TMOD=0x09;//timer0 in 16 bit mode with gate enable
TR0=1;//timer run enabled
TH0=0x00;
TL0=0x00;
P3|=0x04;//setting pin P3.2
while(1)
{
get_range();
delay(2);
}
}
// ****************************************************** // Project: Ultrasonic sensor using 8051 // Author: Code Bloges // Module description: Operate ultrasonic sensor // ****************************************************** #include<REGX52.h> #define port P2 #define dataport P0 int cms; sbit trig=P3^5; sbit rs=port^0; sbit rw=port^1; sbit e=port^2; void delay(unsigned int msec) { int i,j; for(i=0;i<msec;i++) for(j=0;j<1275;j++); } void lcd_cmd(unsigned char item) // Function to send command to LCD { dataport = item; rs= 0; rw=0; e=1; delay(1); e=0; return; } void lcd_data(unsigned char item) // Function to send data to LCD { dataport = item; rs= 1; rw=0; e=1; delay(1); e=0; return; } void lcd_data_string(unsigned char *str) // Function to send string to LCD { int i=0; while(str[i]!='\0') { lcd_data(str[i]); i++; delay(1); } return; } void send_pulse(void) { TH0=0x00;TL0=0x00; trig=1; //Sending trigger pulse delay(5); //Wait for about 10us trig=0; //Turn off trigger } unsigned int get_range(void) { long int timer_val; send_pulse(); while(!INT0); //Waiting until echo pulse is detected while(INT0); //Waiting until echo pulse changes its state timer_val=(TH0<<8)+TL0; lcd_cmd(0x81); lcd_data_string("output:"); lcd_cmd(0x8a); if(timer_val<38000) { cms=timer_val/59; if (cms!=0) { lcd_data(cms+48); } } else { lcd_cmd(0x06); lcd_data_string("Object out of range"); } return cms; } void main() { lcd_cmd(0x38); lcd_cmd(0x0c); delay(2); lcd_cmd(0x01); delay(2); lcd_cmd(0x81); delay(2); lcd_data_string("start"); delay(20); TMOD=0x09;//timer0 in 16 bit mode with gate enable TR0=1;//timer run enabled TH0=0x00; TL0=0x00; P3|=0x04;//setting pin P3.2 while(1) { get_range(); delay(2); } }
Further Reading suggestions:
You may also like,
- Interfacing keypad with 8051
- Interfacing DAC with 8051
- Interfacing with UART of 8051 controller
- Interfacing SPI communication with 8051
- 8051 Displaying Custom Characters on LCD
- 8051 Graphical LCD
- RTC interfacing using I2C in 8051
- Interfacing GPS Modu with 8051
- Interfacing GSM Module with 8051
- Interfacing PWM in 8051
- Interfacing keypad with 8051
- Interfacing DAC with 8051
- Interfacing with UART of 8051 controller
- Interfacing SPI communication with 8051
- 8051 Displaying Custom Characters on LCD
- 8051 Graphical LCD
- RTC interfacing using I2C in 8051
- Interfacing GPS Modu with 8051
- Interfacing GSM Module with 8051
- Interfacing PWM in 8051
No comments:
Post a Comment