Aim:
This tutorial is for beginners in the field of microcontroller. In this
case the microcontroller is AT89C51, a reprogrammable derivative of
8051. This purpose of this tutorial is to familiarize with the use of
push button switch with the microcontroller.
This tutorial is for beginners in the field of microcontroller. In this
case the microcontroller is AT89C51, a reprogrammable derivative of
8051. This purpose of this tutorial is to familiarize with the use of
push button switch with the microcontroller.

Description:
Fig. 1 shows how to interface the switch to microcontroller. A simple switch has an open state and closed state. However, a microcontroller needs to see a definite high or low voltage level at a digital input. A switch requires
a pull-up or pull-down resistor to produce a definite high or low
voltage when it is open or closed. A resistor placed between a digital
input and the supply voltage is called a “pull-up” resistor because it
normally pulls the pin’s voltage up to the supply.
Fig. 1 Interfacing switch to Microcontroller
We now want to control the LED by using switches in 8051 Development board. It works by turning ON a LED & then turning it OFF when switch is going to LOW or HIGH
Fig. 1 shows how to interface the switch to microcontroller. A simple switch has an open state and closed state. However, a microcontroller needs to see a definite high or low voltage level at a digital input. A switch requires
a pull-up or pull-down resistor to produce a definite high or low
voltage when it is open or closed. A resistor placed between a digital
input and the supply voltage is called a “pull-up” resistor because it
normally pulls the pin’s voltage up to the supply.
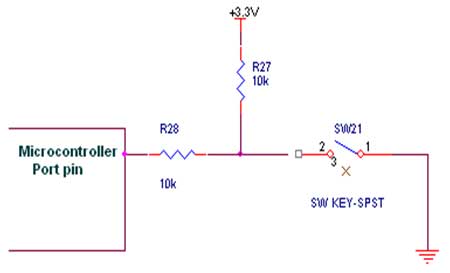
Fig. 1 Interfacing switch to Microcontroller
We now want to control the LED by using switches in 8051 Development board. It works by turning ON a LED & then turning it OFF when switch is going to LOW or HIGH
Block Diagram

Schematic

Code
// ****************************************************
// Project: Interfacing single switch to 8051
// Author: Code Bloges
// Module description: Operate array of LED's
// ****************************************************
#include<reg51.h>
#define ldata P2
sbit LED = P1^0; // define Port2 pin as an LED
sbit Switch = P3^2; // define Port1 pin as Switch
void delay(int);
// Init CCT function
void cct_init(void)
{
P0 = 0x00; // Make all pins zero
P1 = 0x00; // Make all pins zero
P2 = 0x00; // Make all pins zero
P3 = 0x00; // Make all pins zero
}
void main()
{
while(1) // infinite loop
{
if(Switch==0)
{
LED = 1; // toggle LED
}
else
{
LED = 0;
}
}// end of while loop
} // end of main
// **************************************************** // Project: Interfacing single switch to 8051 // Author: Code Bloges // Module description: Operate array of LED's // **************************************************** #include<reg51.h> #define ldata P2 sbit LED = P1^0; // define Port2 pin as an LED sbit Switch = P3^2; // define Port1 pin as Switch void delay(int); // Init CCT function void cct_init(void) { P0 = 0x00; // Make all pins zero P1 = 0x00; // Make all pins zero P2 = 0x00; // Make all pins zero P3 = 0x00; // Make all pins zero } void main() { while(1) // infinite loop { if(Switch==0) { LED = 1; // toggle LED } else { LED = 0; } }// end of while loop } // end of main
Downloads:
The code was compiled in Keil uvision4 and simulation was made in Proteus v7.7.
To download code and proteus simulation click here.
Further Reading suggestions:
You may also like,
- Interfacing keypad with 8051
- Interfacing DAC with 8051
- Interfacing with UART of 8051 controller
- Interfacing SPI communication with 8051
- 8051 Displaying Custom Characters on LCD
- 8051 Graphical LCD
- RTC interfacing using I2C in 8051
- Interfacing ultrasonic sensor with 8051
- Interfacing GPS Modu with 8051
- Interfacing GSM Module with 8051
- Interfacing PWM in 8051
- Interfacing keypad with 8051
- Interfacing DAC with 8051
- Interfacing with UART of 8051 controller
- Interfacing SPI communication with 8051
- 8051 Displaying Custom Characters on LCD
- 8051 Graphical LCD
- RTC interfacing using I2C in 8051
- Interfacing ultrasonic sensor with 8051
- Interfacing GPS Modu with 8051
- Interfacing GSM Module with 8051
- Interfacing PWM in 8051
No comments:
Post a Comment